Which of the following is not an arithmetic operator
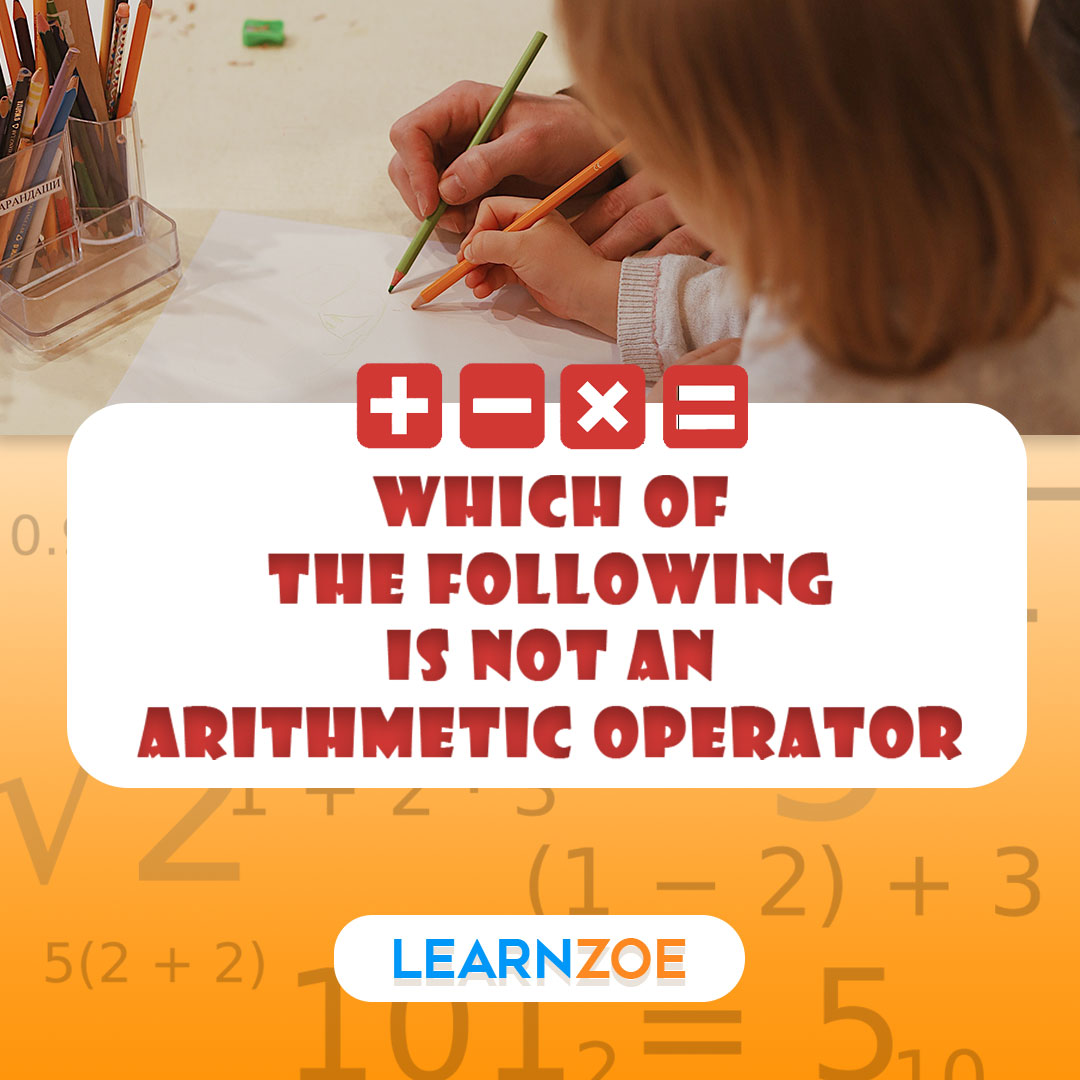
Introduction to Arithmetic Operators
In programming, arithmetic operators are the backbone of any calculation. They are in charge of doing math tasks like addition, subtraction, multiplication, and division of numbers.
Overview of Arithmetic Operators
Definition of arithmetic operators
Arithmetic operators are symbols used in programming languages to perform mathematical calculations. They bridge the human mind and the computer’s logic system, allowing programmers to create complex algorithms.
Examples of arithmetic operators
The most common arithmetic operators include “+” for addition, “-” for subtraction, “*” for multiplication, and “/” for division. Other operators include “%” for modulus (remainder after division) and “++” or “–” for increment or decrement, respectively.
Explanation of addition, subtraction, multiplication, and division
Addition (+): This operator adds two numbers together. For example, 5 + 3 equals 8.
Subtraction (-): This operator subtracts one number from another. For example, 5 – 3 equals 2.Multiplication (*): This operator is used to multiply two numbers together. For example, 5 * 3 equals 15. Division (/): This operator divides one number by another. For example, 15 / 3 equals 5.
Identification of which operator is not an arithmetic operator
The logical operator (!=), also known as “not equal to,” does not fall under the category of arithmetic operators. It’s a comparison operator used to compare two values. For example, if a != b, it means that a is not equal to b.
Here’s a quick overview:
OperatorTypeExample
+ Arithmetic 5 + 3 = 8
– Arithmetic 5 – 3 = 2
* Arithmetic 5 * 3 = 15
/ Arithmetic 15 / 3 = 5
!= Logical If a != b (a is not equal to b)
The addition operator (+) is one of the fundamental arithmetic operators used in mathematics and computer programming. It is commonly used to perform addition, which combines two or more numbers to find their sum. When applied to numbers, the addition operator adds the values together and returns the result. For example, 2 + 3 equals 5.
The addition operator is versatile and can be used with different data types, including integers, floating-point numbers, and even strings. In programming languages like JavaScript and Python, the addition operator can concatenate strings by joining them together. For instance, “Hello” + “World” would result in “HelloWorld”.
However, it is essential to note that the addition operator is unsuitable for all data types. It cannot be used with boolean values or non-numeric data types such as arrays or objects. Attempting to use the addition operator with incompatible data types may result in errors or unexpected behavior.
Other operators, such as logical operators or string concatenation operators, should be used to perform operations on non-numeric data types or boolean values.
The addition operator (+) is a powerful tool for performing arithmetic operations and string concatenation. It allows for the combination of numeric values and the joining of strings. However, it should not be used with boolean values or non-numeric data types.
The subtraction operator (-) is not an arithmetic operator. It is used to subtract one value from another in mathematical calculations. While it is commonly associated with arithmetic operations, it does not fall under the category of arithmetic operators.
Explanation of the Subtraction Operator (-)
Arithmetic operators are symbols or characters used in mathematical calculations to perform addition, subtraction, multiplication, division, and other operations. However, the subtraction operator (-) is not considered an arithmetic operator because it only operates subtraction.
When used in a mathematical expression, the subtraction operator subtracts the value on its right side from its left side. For example, in the expression 5 – 2, the value 2 is subtracted from 5, resulting in a value of 3.
It is important to note that the subtraction operator can be used with different data types, such as integers, floating-point numbers, and even strings. In programming languages, the behavior of the subtraction operator may vary depending on the data types involved.
In summary, while the subtraction operator (-) is commonly used in arithmetic calculations to perform subtraction, it is not classified as an arithmetic operator. It serves the specific purpose of subtracting one value from another. It is an essential component of mathematical expressions and programming languages.
The multiplication operator (*) is one of the fundamental arithmetic operators used in mathematics and programming. It is used to perform the operation of multiplication, which involves multiplying two numbers together to obtain their product.
Explanation of the Multiplication Operator (*)
The multiplication operator (*) is used to multiply two numbers and obtain their product. It is commonly used in mathematical equations and programming languages to calculate multiplication. For example, if you want to find the product of 5 and 3, use the multiplication operator: 5 * 3 = 15.
In computer languages like Python, JavaScript, and C++, the multiplication operator can be used with variables and expressions in multiple ways. It allows you to perform calculations using variables instead of specific numbers. For example, suppose you have two variables, x, and y, and want to find their product. In that case, you can use the following multiplication operator: x * y.
It is important to note that the multiplication operator (*) is not the only arithmetic operator available. There are several other arithmetic operators, including addition (+), subtraction (-), and division (/). Each operator performs a different mathematical operation and has its own rules and precedence.
In conclusion, the multiplication operator (*) is used to operate multiplication in mathematics and programming. It allows you to multiply two numbers or variables to obtain their product.
In the world of arithmetic operators, we encounter several familiar ones regularly, such as addition, subtraction, multiplication, and division. However, not all operators fall into the category of arithmetic operators. One operator that does not belong to this group is the division operator.
Explanation of the Division Operator (/)
With the symbol “/,” you can split one number by another. It figures out the quotient, which is the result of the split. For example, if we use the division operator to split 10 by 2, the answer is 5.
While division is a fundamental mathematical operation, it is not classified as an arithmetic operator. Arithmetic operators primarily perform mathematical calculations involving addition, subtraction, multiplication, and sometimes exponentiation. These operators are used to manipulate numerical values and perform basic mathematical operations.
On the other hand, the division operator is more specific in its purpose and is used exclusively for dividing numbers. It does not encompass the broader range of operations that arithmetic operators typically cover.
In summary, while addition, subtraction, multiplication, and exponentiation are all considered arithmetic operators, the division operator is a specialized operator solely dedicated to dividing numbers.
By understanding the distinction between arithmetic and division operators, we can better grasp their respective roles and functionalities in mathematical calculations.
Modulo Operator
Explanation of the Modulo Operator (%)
The modulo operator is a piece of math that returns the remainder of a division. Usually, the sign “%” is used to show it. The modulo operator is not an arithmetic operation. Adding, subtracting, multiplying, and dividing are.
In a modulo process, the divisor divides the dividend and returns the remainder. For example, if we use the modulo operator to split 10 by 3, the result is 1 since 10 divided by 3 is 3, with a remainder of 1.
There are many ways to use the modulo function in programming and math. It is often used to determine if a number is even or odd since the rest will be 0 for even numbers and 1 for odd numbers. It can also be used to wrap values within a specific range or to go through a set of values in order.
It’s important to remember that the modulo function works in a certain way with negative numbers. In most computer languages, the sign of the result depends on the sign of the dividend.
In conclusion, arithmetic operators include addition, subtraction, multiplication, and division. However, the modulo operator (%) is a unique operator that gives the remainder of a division. It can find parity and cycle through numbers in programming and math.
In computer languages, the increment operator is a type of arithmetic operator that is often used. It is used to make a variable’s number go up by one. But it’s important to remember that the increase operator is not a math operator.
Explanation of the Increment Operator (++)
The increment operator, denoted by ++, is used to increase the value of a variable by one. It can be applied to both integer and floating-point variables. When the increment operator is used as a prefix (++x), the value of x is incremented before it is used in an expression. On the other hand, when it is used as a postfix (x++), the value of x is incremented after it is used in an expression.
For example, if x = 5, ++x would increase x to 6 before it is used in subsequent calculations. Similarly, x++ would result in x being used as 5 in any calculations and then be incremented to 6.
It is essential to understand that the increment operator is not considered an arithmetic operator because it performs no mathematical operations independently. Instead, it is used to modify the value of a variable.
In conclusion, while the increment operator (++x or x++) is commonly used in programming languages to increase the value of a variable by one, it is not classified as an arithmetic operator.
The decrement operator is a unary arithmetic operator subtracting 1 from its operand. The symbol represents it “–.” This operator is commonly used in programming languages such as C, C++, Java, and JavaScript.
Explanation of the Decrement Operator (–)
With the decrement function, the value of a variable goes down by 1. It can be used with integers and floating-point numbers, both types of math data. The decrement operator is called the pre-decrement operator when it comes before a variable. It is called the post-decrement operator when it comes after a variable.
For example, if we have a variable “x” with an initial value of 5, using the pre-decrement operator like “–x” will subtract 1 from “x” before using its value in an expression. So, the new value of “x” will be 4.
On the other hand, using the post-decrement operator like “x–” will take away 1 from “x” after using its value in an expression. So, “x” will still have a value of 5 until it is used again.
It is important to remember that the decrement operator only works with variables and not with numbers or literals. Also, using the decrement operator more than once in a single statement can lead to unexpected effects and should be avoided for clarity and readability.
In conclusion, the decrement operator is not an arithmetic operator; it is a unary operator used to reduce the value of a variable by 1. It is a necessary part of computer languages for loops and if/then statements.
After learning about the different arithmetic operators and what they do, it is clear that the assignment operator (=) is the only one not an arithmetic operator. The assignment operator gives a variable a value. The other operators (+, -, *, and /) are used for math tasks.
Identifying the Non-Arithmetic Operator
Knowing the difference between arithmetic operators and other operators is essential when working with programming languages or math processes. The arithmetic operations, such as (+), (-), (*), and (/), are made for math calculations like adding, subtracting, multiplying, and dividing. These functions change the values of numbers.
On the other hand, the assignment operator (=) is not an arithmetic operator. Its purpose is to assign a value to a variable. For example, in programming, you can use the assignment operator to assign a value to a variable like this: “x = 5”. This statement assigns the value 5 to the variable x.
It’s important to remember that even though the assignment operator may sometimes work with numbers, its primary purpose is not to do math calculations. Instead, a program uses a data storage method to keep and work on information.
Ultimately, the assignment operator (=) is not one of the given math operators. It is essential to understand this difference when working with programming languages or mathematical processes.